Artist对象
Artist对象
python科学计算第二版 张若愚
matplotlib的基本图像是这样的,所有的绘图元素都继承自Artist类,在matplotlib画出的图上看到的一个个元素,实际上就是这些对象。
总体上,这些对象可以分为简单类型和容器类型两种,简单的Artist对象是标准的绘图元件,例如plot()
方法生成的Line2D对象,add_patch()
方法生成的Rectangle对象,text()
方法生成的Text对象等等。更高级的方法可能会绘制出更复杂的元素,但本质上都是这些基本对象的组合。
容器类型可包含多个Artist对象,使他们组织成一个整体,例如Axis,Axes,Figure等,这些基本绘图元素都储存在容器对象中,比如绘制的Axes对象都储存在Figure.axes列表中,Axes绘制的Line2D对象都储存在Axes.lines列表中,绘制的Rectangle对象都储存在Axes.patches列表中,而轴axis又是Axes下面的一个子容器Axes.xaxis,轴上又有Axes.xaxis.label标签等元素。
通过这种一层层的嵌套组合,最终构成了一幅绘图。要清楚的就是大家都是对象,同时都继承自Artist基类,所以有一些一致的接口,比如set_*()
和get_*()
,分别设置和获取自己的属性。比如如果想让某个东西看不见,方法都是一样的*.set_visible(False)
。
有时候很多看似复杂的绘图要素,往下一层层分析,发现他某个特点可能是一个很基本的对象,比如Text,这时候就可以用Text对象的接口去对这个属性进行修改。同时,matplotlib提供了一些更高级的接口,比如可以用Axes.set_xticks, Axes.set_xticklabels, Axes.tick_params()
去修改标签的属性,但要知道,这些属性并不是Axes所具有的,而是Axes下子容器所拥有的属性,这里只是语法糖。认识到这一点,对matplotlib的认识就会清晰很多,很多命令就都不需要特意去记忆了。
一个例子
1 | # create figure |
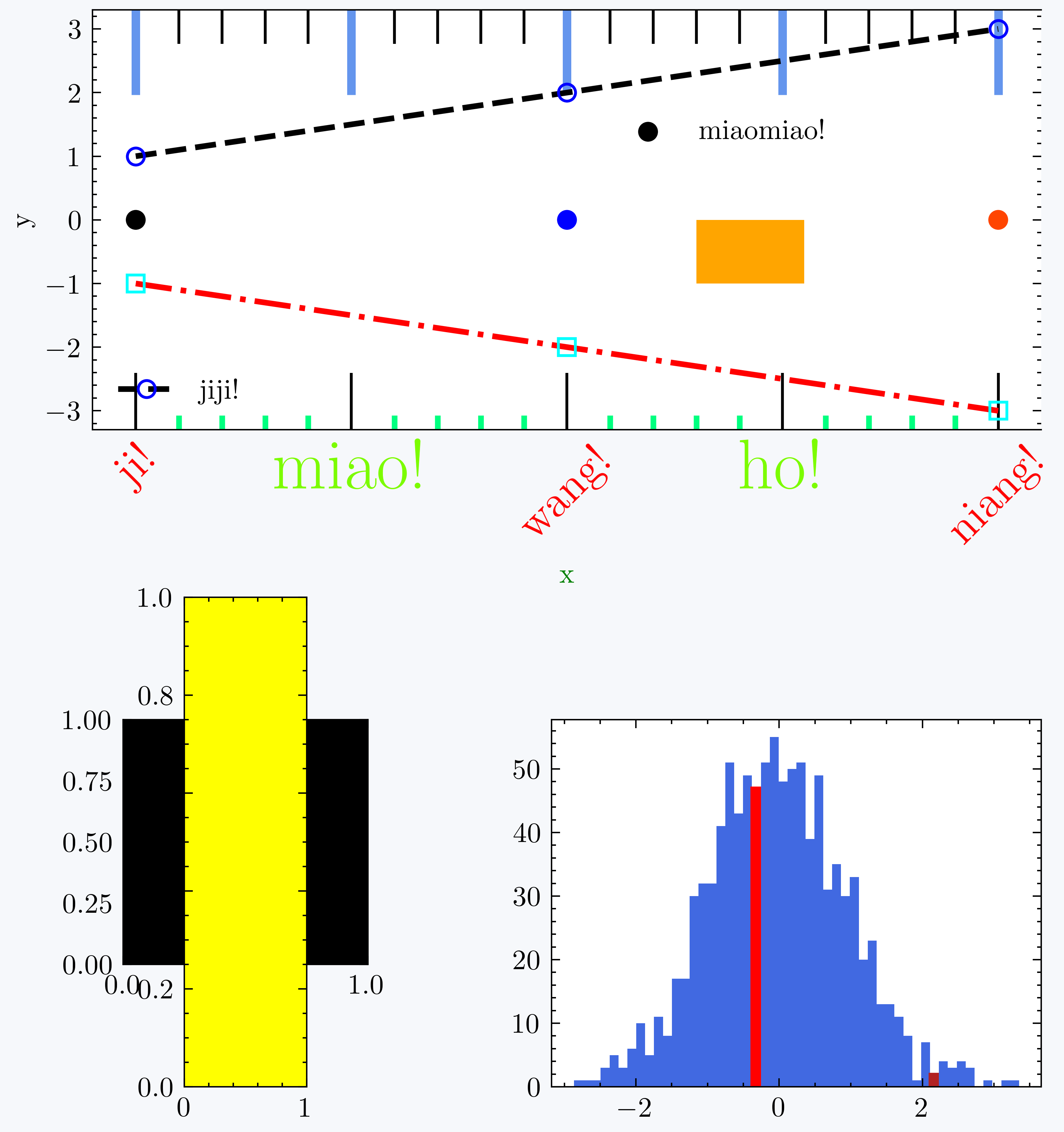
一些评论
plt.figure()
创建一个Figure对象,fig.add_axes()
创建一个Axes对象,参数是一个形如[x0, y0, width, height]的列表,各个值都是Figure对象长度和宽度的相对值。在构成图表的各种Artist对象中,最上层的对象是Figure,它包含组成图表的所有元素。当调用add_subplot()
或add_axes()
方法往图表中添加子图时,这些子图都将添加到Figure对象的axes属性中:
1 | print(fig.axes) |
1 | [<AxesSubplot:xlabel='x', ylabel='y'>, <Axes:>, <Axes:>, <Axes:>] |
Axes容器是matplotlib的核心,它包含了组成图表的众多Artist对象,并且有许多方法函数帮助创建和修改这些对象。和Figure容器一样,它拥有一个patch属性作为背景,当它是笛卡尔坐标时,patch属性是一个Rectangle对象;当它是极坐标时,patch属性是一个Circle对象。
当然,这里matplotlib提供了一个语法糖,Axes本身也有facecolor属性,对它进行修改就想当于修改patch属性的facecolor属性。
大体上来讲,Axes对象包含两类绘图元素,一类是plot()
方法绘制出的Line2D对象,它们都被储存在Axes对象的lines属性列表中;另一类是比如绘制柱状图的bar()
函数与直方图的hist()
函数,这些函数将创建一个Patch对象的列表,每个元素都是从Patch类派生的Rectangle对象,所创建的对象都被添加进了Axes对象的patches属性中。
ax.plot()
绘制曲线,返回表示该曲线的Line2D对象,返回值是只有一个对象的列表(因为可以传递多组X-Y轴的数据给plot()
,同时绘制多条曲线)。
ax.lines
是一个包含所有曲线的列表,每用ax.plot()
绘制一条曲线,所创建的Line2D对象就会添加到该列表中。要删去某个曲线,删去列表中的对应元素即可。
1 | print(line_1[0]) |
1 | [<matplotlib.lines.Line2D object at 0x7f91f2f8f370>] |
图例接受两个参数,handles是一个Artist对象的列表,labels是对应的标签列表。
ax.set_xlabel()
可以设置x轴的标题,但这实际上是matplotlib提供的语法糖,Axes本身并没有xlabel属性:
1 | print(ax.xaxis) |
1 | XAxis(37.8,18.900000000000002) |
Axes对象的xaxis属性是一个XAxis对象,XAxis对象的label属性是一个Text对象,Text对象的_text属性为设置的字符串。由于它们都是从Artist类继承过来的,所以可以像上面这样,设置Text对象的属性:
1 | ax.get_xaxis().get_label().set_color("green") |
也可以像上面一样,用set()
一次设置多个属性。
想要查看某个Artist对象的所有属性名及其对应的值,可以使用
1 | plt.getp(ax.xaxis.label) |
Axis容器包括坐标轴上的刻度线、刻度文本、坐标网格以及坐标轴标题等内容。刻度包括主刻度和副刻度,分别通过get_major_ticks()
和get_minor_ticks
方法获得。每个刻度线都是一个XTick或YTick对象,它包括实际的刻度线和刻度文本。为了方便访问刻度线和文本,Axis对象提供了get_ticklabels()
和get_ticklines()
方法来直接获得刻度线文本和刻度线,刻度线是一个Line2D对象,刻度线文本是一个Text对象。
当然,这样修改太复杂了,matplotlib提供了Axes的tick_prams()
方法,直接对标签和刻度的属性做出修改。