使用console_scripts打包python代码
使用console_scripts打包python代码
我们写了一个Python包,比如包的名字叫cat,现在想提供一个命令行工具,用户只要在命令行中输入cat+各种参数就可以运行我们的包,比如输入cat call
返回miaow! ,进一步地,如果在后面再输入一个数字,则可控制cat叫的次数,比如cat call 2
. 输入cat draw
画出一只小猫的漫画. 我们可以用argparse库实现对参数的解析,并将其绑定到不同函数上,用setup.py中的console_scripts参数来实现命令行工具的打包. 帝国理工的PyFR项目是用这一套方案来进行开发的,安装好PyFR包以后,只要在命令行中输入
1 | pyfr run mesh.pyfrm configuration.ini |
就可以展开模拟了,其中mesh.pyfrm
是网格数据,configuration.ini
是模拟的输入文件. 像是这种配置文件.. hexo采用的是YAML格式,Vscode是JSON格式,julia的项目配置文件是TOML,python的pdm也是TOML,看起来TOML好像更方兴未艾一点..
argparse
argparse这个库使用的基本想法就是维护一个ArgumentParser对象,通过这个对象的各种方法来实现对命令行参数的解析,并将最终解析的结果保存到一个Namespace对象中. 像是Lammps其实也是维护了一个lammps对象,并通过这个对象定义的方法开展模拟的. 我们可以用.
运算符来获取Namespace对象中的参数.
1 | auto lammps = new LAMMPS(argc, argv, lammps_comm); |
所以
1 | # cat.py |
在命令行中输入
1 | python cat.py -n jet --lovefood meat |
输出为
Namespace(lovefood='meat', name='jet')
我们也可以通过subparser实现对函数的调用,subparser可以继续定义该函数的参数,通过subparser的set_defaults
方法绑定对应函数,比如下面的程序,我们可以执行
1 | python cat.py call 3 |
输出为
miaow! miaow! miaow!
这里的逻辑是当给定参数call后,call对应的sub_parser -> ap_call会继续对后面的参数按照add_argument
方法的指定进行检索.
1 | # cat.py |
console_scripts
现在我们想把它打包成一个包,并提供相应的命令行工具,希望安装好cat包以后,直接在命令行里执行cat call 3
就可以实现之前的功能了,我们可以借助setuptools中提供的console_scripts入口实现这一想法. 按照下述的方式组织cat包.
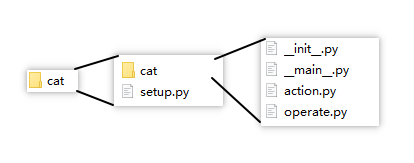
其中各文件内容为,这个console_scripts就相当于打包了__main__.py中的main函数.
1 | # setup.py |
1 | # __init__.py |
1 | # __main__.py |
1 | # action.py |
1 | # operate.py |
使用conda新建一个虚拟环境,在setup.py目录下执行pip install .
,即可安装cat包,在命令行里测试一下
